Introduction
This is a simple billing project which was developed using C# & SQL Server 2005. You can use it for all type of invoice/Bill preparation after small modification.Download Project
Download SQL Script
Video in Youtube
Create Database
There are two ways to create a database.
1. SQL Management Studio Query Analyser.
2. SQL Management Studio Visual Tool.
The following sql script create a database with default option using SQL Management Studio Query Analyser.
CREATE DATABASE [MyBill]
(where MyBill is a name of database)
Open SQL Server Management Studio Express and execute the above mentioned query.
Create a database using SQL Server Management Studio Express
Right Click on Databases.
Select New Database... from the popup
Type your database name(Eg. "MyBill")
Select "OK"
Table Creation
Table Name | Purpose |
---|---|
CompanyInfo | Store the Company name & Address, it will be used in Invoice |
PrMaster | Store the Product details such as Product code, description, Rate, Unit etc. |
InvoiceMain | Store the invoice details such as Invoice No., Date, Customer Name & Address, |
InvoiceSub | Store the product details for corresponding invoice. |
Copy the following script and paste in SQL Server Management studio Query Analyser. Press "F5" or Click "Run" button in the Tool bar.
Use MyBill go CREATE TABLE [dbo].[InvoiceMain]( [InvId] [int] IDENTITY(1,1) NOT NULL, [InvoiceNo] [int] NULL, [InvoiceDate] [datetime] NULL, [InvoiceCustomer] [varchar](200) NULL, [InvoiceAddress] [varchar](500) NULL, [InvoiceCustomerId] [varchar](50) NULL, [InvoiceChequeNo] [varchar](25) NULL, [InvoiceBank] [varchar](100) NULL, [InvChequeDate] [datetime] NULL, [InvNetAmount] [numeric](18, 2) NULL, [InvTaxNetAmount] [numeric](18, 2) NULL, CONSTRAINT [PK_InvoiceMain] PRIMARY KEY CLUSTERED ( [InvId] ASC ) ON [PRIMARY] ) ON [PRIMARY] GO SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO CREATE TABLE [dbo].[InvoiceSub]( [RowId] [int] IDENTITY(1,1) NOT NULL, [InvId] [int] NULL, [PrId] [int] NULL, [PrQty] [numeric](18, 2) NULL, [PrRate] [numeric](18, 2) NULL, [PrTaxPercent] [numeric](18, 2) NULL, CONSTRAINT [PK_InvoiceSub] PRIMARY KEY CLUSTERED ( [RowId] ASC ) ON [PRIMARY] ) ON [PRIMARY] GO SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO CREATE TABLE [dbo].[CompanyInfo]( [CompanyId] [int] IDENTITY(1,1) NOT NULL, [CompanyName] [varchar](100) NULL, [CompanyAddress1] [varchar](100) NULL, [CompanyAddress2] [varchar](100) NULL, [CompanyAddress3] [varchar](100) NULL, [CompanyPINCode] [varchar](50) NULL, [CompanyPhone1] [varchar](100) NULL, [CompanyTNGST] [varchar](50) NULL, [CompanyCST] [varchar](50) NULL, [CompanyTIN] [varchar](50) NULL, CONSTRAINT [PK_CompanyInfo] PRIMARY KEY CLUSTERED ( [CompanyId] ASC ) ON [PRIMARY] ) ON [PRIMARY] GO SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO CREATE TABLE [dbo].[PrMaster]( [PrId] [int] IDENTITY(1,1) NOT NULL, [PrCode] [varchar](50) NULL, [PrDesc] [varchar](500) NULL, [PrUnit] [varchar](50) NULL, CONSTRAINT [PK_PrMaster] PRIMARY KEY CLUSTERED ( [PrId] ASC ) ON [PRIMARY] ) ON [PRIMARY] GO
Insert data into Companyinfo Table
INSERT INTO [CompanyInfo] ([CompanyName] ,[CompanyAddress1] ,[CompanyAddress2] ,[CompanyAddress3] ,[CompanyPINCode] ,[CompanyPhone1] ,[CompanyTNGST] ,[CompanyCST] ,[CompanyTIN]) VALUES ('My Company', 'Address1', 'Address2', 'Address3', 'PIN Code', 'Phone No', 'TNGST No', 'CST No', 'TIN' ) go Select * from CompanyInfo
Insert Data in Product Master
Please refer the screen structure. I have designed this application in VS 2010 with C# Code. Below mentioned screen used to create Product.
Saved data in SQL Server.
Please refer screen structure for Invoice/Bill.
Invoice List
Add - Create New Invoice.
Delete - Delete the selected Invoice.
Close - Close Invoice List.
Print - Print the selected Invoice.
Close - Close Invoice List.
Print - Print the selected Invoice.
Report Designing - Crystal Report.
Now I am going to design invoice/Billing application in below mentioned format using Crystal Report.Download Crystal report for Visual studio 2010
Add Crystal Report
Select Project Menu->Add New Item->Visual C# Items->Crystal ReportsType the Report Name(MyInvoice.rpt)
Select "As a Blank Report"
Report Sections
Connect with Data source
Select View Menu->Server Explorer(Refer below screen)Follow the images for data source connections.
Add table schema to Dataset
Drag & drop the "PrintInvoice" stored Procedure with "Invoicedataset.xsd"(Refer below screen)PrintInvoice is a Stored Procedure which returns the necessary records for print the Invoice.
Place the field in Crystal Report.
Swith to MyInvoice.rpt Design mode.Open Field Explorer.(If Field Explorer not visible Go to View Menu View->Other Windows->Document Outline)
Paper size & Margin Settings
Refer the below screen for Paper size & Margin settings.
Report Design
Place the fields in Report Area
Place the fields in Report(Refer the Image)Preview the Report
Add new form and Place Crystal report viewer and name it CRV.Change the DOCK Property of Crystal report viewer to Fill(Refer screen)
Switch to design view of frmInvoiceList.cs. Double click and write the following code in click Event
Refer the screen for Print the invoice.
private void btnPrint_Click(object sender, EventArgs e) { if (lstMain.SelectedItems.Count == 0) { MessageBox.Show("Please select the invoice..."); return; } DataTable dt = new DataTable(); string InvId; InvId = this.lstMain.SelectedItems[0].SubItems[6].Text; dt = Invoice.InvoicePrint(InvId); frmReportShow f = new frmReportShow(); rptinvoice rpt = new rptinvoice(); rpt.SetDataSource(dt); f.CRV.ReportSource = rpt; f.ShowDialog(); }
Print the Invoice(Refer Screen)
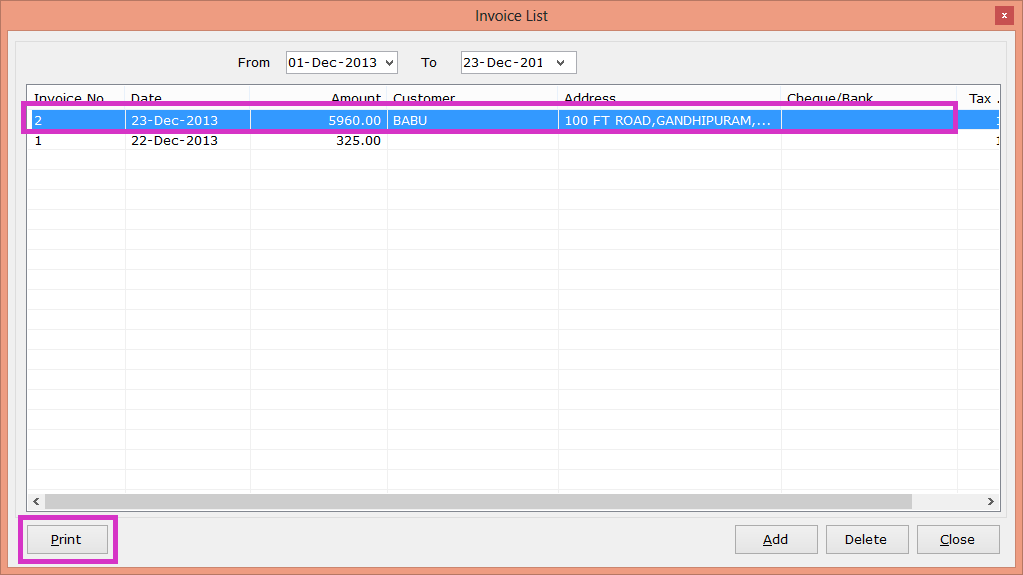
Invoice Output
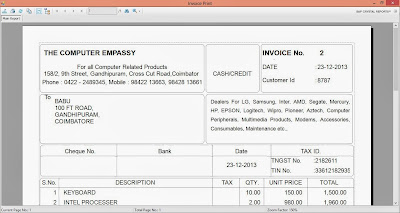
Points to Remember
Just look at below screen.
Script of PrintInvoice stored Procedure
ALTER Proc [dbo].[PrintInvoice](@InvId int) as SELECT dbo.InvoiceMain.InvId, dbo.InvoiceMain.InvoiceNo,dbo.InvoiceMain.InvoiceDate, dbo.InvoiceMain.InvoiceCustomer, dbo.InvoiceMain.InvoiceAddress, dbo.InvoiceMain.InvoiceCustomerId, dbo.InvoiceMain.InvoiceChequeNo,dbo.InvoiceMain.InvoiceBank, dbo.InvoiceMain.InvChequeDate, dbo.InvoiceMain.InvNetAmount, dbo.InvoiceMain.InvTaxNetAmount,dbo.InvoiceSub.RowId, dbo.InvoiceSub.PrId,dbo.InvoiceSub.PrQty,dbo.InvoiceSub.PrRate, dbo.InvoiceSub.PrTaxPercent,dbo.PrMaster.PrCode, dbo.PrMaster.PrDesc,dbo.PrMaster.PrUnit, dbo.CompanyInfo.CompanyName, dbo.CompanyInfo.CompanyAddress1, dbo.CompanyInfo.CompanyAddress2,dbo.CompanyInfo.CompanyAddress3, dbo.CompanyInfo.CompanyPINCode, dbo.CompanyInfo.CompanyPhone1, dbo.CompanyInfo.CompanyTNGST, dbo.CompanyInfo.CompanyCST, dbo.CompanyInfo.CompanyTIN, dbo.AmountToWords(InvNetAmount)+' only.' as Amountinwords FROM dbo.InvoiceMain INNER JOIN dbo.InvoiceSub ON dbo.InvoiceMain.InvId = dbo.InvoiceSub.InvId INNER JOIN dbo.PrMaster ON dbo.InvoiceSub.PrId = dbo.PrMaster.PrId CROSS JOIN dbo.CompanyInfo where dbo.InvoiceMain.InvId=@InvId order by rowIdThe field InvId(Identity Column) is a primary key of InvoiceMain Table.
The field InvId is a Foreign key of InvoiceSub Table which used to create ONE TO MANY relationship between the tables.
The PrintInvoice stored Procedure used to print the invoice. When you execute this procedure it should return some records. If it is return Zero record, the blank report will open.
In invoice no 1, there are two items are used.
In invoice no 2, there are three items are used.
ER Diagram for Invoice Print
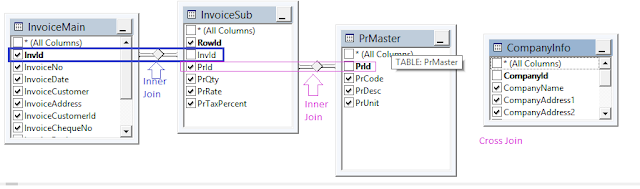
The CompanyInfo table must have only one record. If it have more than one record, the duplicate records will be return byPrintInvoice stored procedure.
The CompanyInfo table is optional. I have stored the Company details in that table. You can directly type(static text) company details in Crystal Report using Text box control. After deployment of the application, you may need to change the company details. If you use the CompanyInfo table, just update the details in Corresponding field in CompanyInfo. It will reflect immediately in the report.
if you use the static text method, you have to again deploy the entire project.
If you want the source code, Please send the mail to mailtobabum@gmail.com
Troubleshoot
So many user post their comments. But they have written, the application returned blank reports. Please run the following query in your Sql Server management studio(Refer Image). All the query should return at least one row. Otherwise you will get the blank report. If any of the query return no records, kindly check whether the table has record or not.
select * from [dbo].[CompanyInfo] select * from [dbo].[PrMaster] select * from [dbo].[InvoiceMain] order by invid select * from [dbo].[InvoiceSub] order by invid SELECT dbo.AmountToWords(InvNetAmount)+' only.' as Amountinwords, dbo.InvoiceMain.InvId, dbo.InvoiceMain.InvoiceNo, dbo.InvoiceMain.InvoiceDate, dbo.InvoiceMain.InvoiceCustomer, dbo.InvoiceMain.InvoiceAddress, dbo.InvoiceMain.InvoiceCustomerId, dbo.InvoiceMain.InvoiceChequeNo, dbo.InvoiceMain.InvoiceBank, dbo.InvoiceMain.InvChequeDate, dbo.InvoiceMain.InvNetAmount, dbo.InvoiceMain.InvTaxNetAmount, dbo.InvoiceSub.RowId, dbo.InvoiceSub.PrId, dbo.InvoiceSub.PrQty, dbo.InvoiceSub.PrRate, dbo.InvoiceSub.PrTaxPercent, dbo.PrMaster.PrCode, dbo.PrMaster.PrDesc, dbo.PrMaster.PrUnit, dbo.CompanyInfo.CompanyName, dbo.CompanyInfo.CompanyAddress1, dbo.CompanyInfo.CompanyAddress2, dbo.CompanyInfo.CompanyAddress3, dbo.CompanyInfo.CompanyPINCode, dbo.CompanyInfo.CompanyPhone1, dbo.CompanyInfo.CompanyTNGST, dbo.CompanyInfo.CompanyCST, dbo.CompanyInfo.CompanyTIN FROM dbo.InvoiceMain INNER JOIN dbo.InvoiceSub ON dbo.InvoiceMain.InvId = dbo.InvoiceSub.InvId INNER JOIN dbo.PrMaster ON dbo.InvoiceSub.PrId = dbo.PrMaster.PrId CROSS JOIN dbo.CompanyInfo
Comments
Please help me sooon...........
thank you so much
Now i can print invoice because of your article
Plzz help me...
ma maill id is dsksaravana@hotmail.com
cloud billing software
SQIAR (http://www.sqiar.com/solutions/technology/tableau) is a leading Business Intelligence company and provides Tableau Software consultancy across United Kingdom and USA.
Thank you so much for this.
its so helpfull
EMAIL ID:nturankar@yahoo.com
I get Invoioce Print Project Run Successfully but it generate Blank report Please help me out.
Thanks.
email id:shumakar07@gmail.com
Pls Help me to fix it.
Thanks in Advance
Download and Install Crystal from
http://downloads.businessobjects.com/akdlm/cr4vs2010/CRforVS_13_0_2.exe
please help me out...
My Email Id : apurvpungliya@gmail.com
Run the following qry. It should return a row.
Select * from CompanyInfo
If no record found, please insert below record.
INSERT INTO [CompanyInfo]
([CompanyName]
,[CompanyAddress1]
,[CompanyAddress2]
,[CompanyAddress3]
,[CompanyPINCode]
,[CompanyPhone1]
,[CompanyTNGST]
,[CompanyCST]
,[CompanyTIN])
VALUES
('My Company',
'Address1',
'Address2',
'Address3',
'PIN Code',
'Phone No',
'TNGST No',
'CST No',
'TIN'
)
Below mentioned qry is final qry. The qry should return at least one row. Otherwise you will get blank report. If your invoice has 2 product, it should return 2 rows and so on.
SELECT dbo.InvoiceMain.InvId,
dbo.InvoiceMain.InvoiceNo,dbo.InvoiceMain.InvoiceDate, dbo.InvoiceMain.InvoiceCustomer,
dbo.InvoiceMain.InvoiceAddress, dbo.InvoiceMain.InvoiceCustomerId,
dbo.InvoiceMain.InvoiceChequeNo,dbo.InvoiceMain.InvoiceBank,
dbo.InvoiceMain.InvChequeDate, dbo.InvoiceMain.InvNetAmount,
dbo.InvoiceMain.InvTaxNetAmount,dbo.InvoiceSub.RowId,
dbo.InvoiceSub.PrId,dbo.InvoiceSub.PrQty,dbo.InvoiceSub.PrRate,
dbo.InvoiceSub.PrTaxPercent,dbo.PrMaster.PrCode,
dbo.PrMaster.PrDesc,dbo.PrMaster.PrUnit,
dbo.CompanyInfo.CompanyName, dbo.CompanyInfo.CompanyAddress1,
dbo.CompanyInfo.CompanyAddress2,dbo.CompanyInfo.CompanyAddress3,
dbo.CompanyInfo.CompanyPINCode, dbo.CompanyInfo.CompanyPhone1,
dbo.CompanyInfo.CompanyTNGST, dbo.CompanyInfo.CompanyCST,
dbo.CompanyInfo.CompanyTIN,
dbo.AmountToWords(InvNetAmount)+' only.' as Amountinwords
FROM dbo.InvoiceMain
INNER JOIN dbo.InvoiceSub ON dbo.InvoiceMain.InvId =
dbo.InvoiceSub.InvId INNER JOIN
dbo.PrMaster ON dbo.InvoiceSub.PrId =
dbo.PrMaster.PrId CROSS JOIN
dbo.CompanyInfo
where dbo.InvoiceMain.InvId=@InvId
order by rowId
Please help me,
My email id is pradeepupadhyay471@gmail.com
i think crystal report is not useful
andymore so many out there software that i can say is fully innovative than crystal report like wisp billing system
Invoice Management Software
I am currently using CloudBooks as Billing Software and completely satisfied with it and suggest to everyone to try it. For More info visit at: - https://www.cloudbooksapp.com/
email:-ankittajpara@gmail.com
Thanks a lot for this post.
Email- ravi29khandelwal@gmail.com
Did you install the Crystal report?
please install it and try again.
Crystal report URL :http://downloads.businessobjects.com/akdlm/cr4vs2010/CRforVS_13_0_2.exe
Assayamu galeykum . Please help me. I downloaded the project and the base , now with the addition of "Invoice" in the "Invoice list" is not added.
email: beima@bk.ru
skype: beiima
Assayamu galeykum . Please help me. I downloaded the project and the base , now with the addition of "Invoice" in the "Invoice list" is not added.
email: beima@bk.ru
skype: beiima
You can visit our official website: http://www.myentry.co.in
GST Supported| Different Invoice templates| Platform Independent| Import/Export Feature
Simple & secure Invoicing though there is no better substitute for Saksham Billing & Invoice Software. You can create & send attractive Invoices with customized designs.
Get all this features in a single kit
For Free Demo Call us at: 8947027625
To know more click here : www.kriscent.in
Or www.saksham.kriscent.in
Or You may also reach us at: info@kriscent.in
Online Billing Software
Thanks for sharing a very interesting article about Invoice POS Billing Application Software. This is very useful information for online blog review readers. Keep it up such a nice posting like this.
From,
Retailmass,
POS Billing Software
Busy Accounting Software App
Billing Software App
GST Billing App
appgst
Billing Software
go gst bill software
Free Inventory Management Software with GST
Inventory Management and Billing Software
Stock Management and Billing Software
Accounting Software
Accounting App
Online Accounting Software
GST Billing Software
Billing Software
Accounting Software India
GST Ready Software
Inventory Management Software
Accounting And Inventory Software
Financial Management
GST Tax Return
GST Tax Filing
Inventory Management
Retail and Restaurant Billing Software
Billing POS Software for Retail and Restaurants
Just Billing Software Distribution Management Software
Distribution Management Software
Free Retail POS
Cloud Restaurant Software
stock software for small business
Are you searching for any inexpensive billing software in Chennai? We are here
Billing Software in Chennai
Restaurant Billing Software in Chennai
Supermarket Billing Software in Chennai
Retail Billing Software in Chennai
Cloud Billing Software in Chennai
Medical Billing Application
Adobe Photoshop Download
SuperAntiSpyWare Pro
PC Helpsoft Driver"